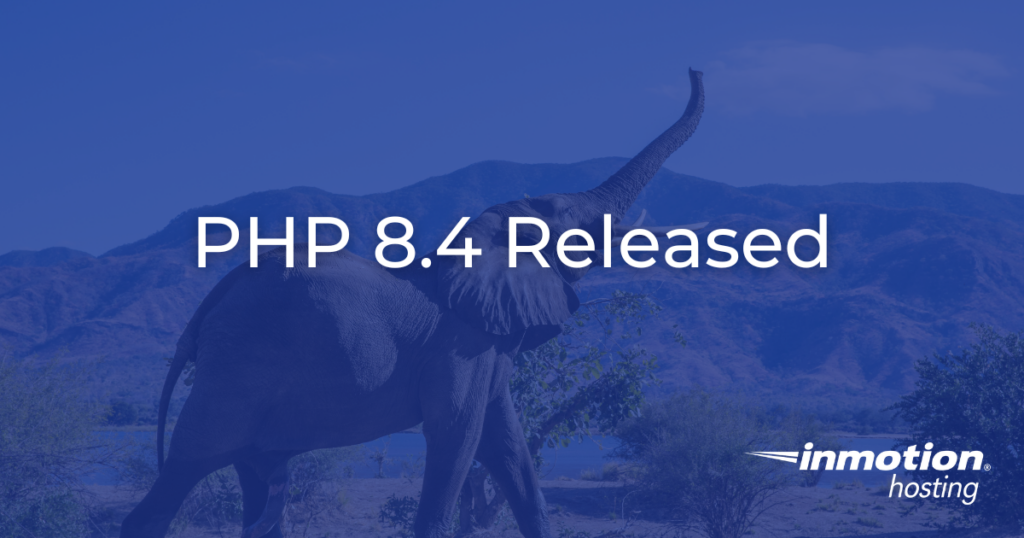
PHP 8.4 was released November 21, 2024. As of this writing, you can use PHP 8.4 on cPanel VPS environments and Dedicated servers by enabling the package in EasyApache. PHP 8.4 will be added to shared servers after a brief period of testing by InMotion’s Engineering team.
What’s new in PHP 8.4?
HTML 5 Compatibility
One of the largest improvements is to the \DOMDocument library, which in previous versions was almost compatible with HTML 4. While HTML 5 has been around for over 16 years, PHP still relied on a shoehorn method of using LIBXML and quiet errors to parse most tags outside of the most basic HTML tags.
In PHP 8.4, the new \Dom\HTMLDocument object allows parsing of all HTML5-compliant tags, and because it’s using the new \Dom\ namespace, remains fully backward-compatible with the old \DOMDocument objects. This means developers can continue to use older code that works, but take advantage of the new features as they develop new products.
Property Hooks
Property Hooks will be a boon to developers, allowing them to skip a lot of the basic “boilerplate” code that was previously needed when creating new classes and objects.
Take for example the following class from the PHP Foundation announcement, which contains a few private members:
//PHP < 8.4
class Locale
{
private string $languageCode;
private string $countryCode;
public function __construct(string $languageCode, string $countryCode)
{
$this->setLanguageCode($languageCode);
$this->setCountryCode($countryCode);
}
public function getLanguageCode(): string
{
return $this->languageCode;
}
public function setLanguageCode(string $languageCode): void
{
$this->languageCode = $languageCode;
}
public function getCountryCode(): string
{
return $this->countryCode;
}
public function setCountryCode(string $countryCode): void
{
$this->countryCode = strtoupper($countryCode);
}
public function setCombinedCode(string $combinedCode): void
{
[$languageCode, $countryCode] = explode('_', $combinedCode, 2);
$this->setLanguageCode($languageCode);
$this->setCountryCode($countryCode);
}
public function getCombinedCode(): string
{
return \sprintf("%s_%s", $this->languageCode, $this->countryCode);
}
}
$brazilianPortuguese = new Locale('pt', 'br');
var_dump($brazilianPortuguese->getCountryCode()); // BR
var_dump($brazilianPortuguese->getCombinedCode()); // pt_BR
In this example, the developer has had to write explicit getter and setter functions for each of the member variables. In PHP 8.4, however, it is much easier:
//PHP 8.4
class Locale
{
public string $languageCode;
public string $countryCode
{
set (string $countryCode) {
$this->countryCode = strtoupper($countryCode);
}
}
public string $combinedCode
{
get => \sprintf("%s_%s", $this->languageCode, $this->countryCode);
set (string $value) {
[$this->languageCode, $this->countryCode] = explode('_', $value, 2);
}
}
public function __construct(string $languageCode, string $countryCode)
{
$this->languageCode = $languageCode;
$this->countryCode = $countryCode;
}
}
$brazilianPortuguese = new Locale('pt', 'br');
var_dump($brazilianPortuguese->countryCode); // BR
var_dump($brazilianPortuguese->combinedCode); // pt_BR
Invoke New Class Methods without Parentheses
In previous versions of PHP, if you wanted to invoke a method of a new class on the same line, the class declaration had to be wrapped in parentheses, like so:
$member = (new ExampleClass($argument))->getMember();
Now, this is more convenient with the new syntax:
$member = new ExampleClass($argument)->getMember();
Implement Your Own Deprecations With the #[\Deprecated] Attribute
You can now use PHP’s built-in deprecation mechanism to alert developers using your code of new ways to accomplish deprecated methods. This will create automatic debug output, similar to deprecations in PHP core.
class MyClass
{
#[\Deprecated(
message: "Doing it wrong, use MyClass::newMethod() instead",
since: "8.4",
)]
public function oldMethod(): string
{
return $oldWay;
}
public function newMethod(): string
{
return $newWay;
}
}
New Array Functions and More
New functions, including array_find(), array_find_key(), array_any(), and array_all() have been introduced to avoid having to write your own searching functions. There are many other new features, be sure to check out the PHP Official Release announcement here.
PHP 8.4 Availability on your InMotion Hosting Server
PHP 8.4 is already available on VPS accounts using cPanel and CWP. Users with root access can use EasyApache for cPanel to install the new version. CWP administrators can install PHP 8.4 using these instructions.
Our system administrators are currently evaluating the new version for inclusion on shared servers, and it will be available in the near future.