Table of Contents
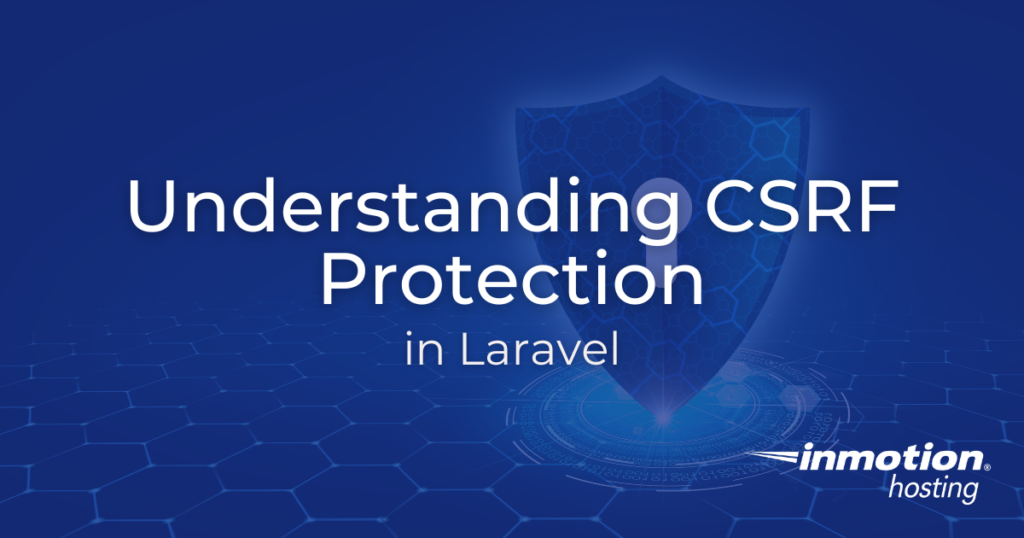
Cross-Site Request Forgery (CSRF) is an attack in which unauthorized commands are transmitted from a user that the web application trusts. Laravel provides robust CSRF protection out of the box to help secure your application from such vulnerabilities. This article will guide you through the basics of CSRF, how Laravel protects against CSRF attacks, and how to implement and manage CSRF protection in your Laravel applications.
- What is CSRF?
- How Laravel Protects Against CSRF
- Implementing CSRF Protection
- Configuring CSRF Token Rotation
- Disabling CSRF Protection for Specific Routes
- Complete Example of CSRF Protection
- Common Issues and Troubleshooting
- Conclusion
What is CSRF?
CSRF (Cross-Site Request Forgery) is an attack that tricks a user into submitting a malicious request. It leverages the fact that a browser automatically includes credentials (like cookies) with each request, enabling an attacker to perform actions on behalf of the user without their consent.
How Laravel Protects Against CSRF
Laravel provides built-in CSRF protection to safeguard your application from such vulnerabilities. Here’s how it works:
- CSRF Tokens: Laravel generates a unique CSRF token for each active user session. This token is then embedded in every form of the application as a hidden field. The server verifies this token on every request to ensure the request is valid.
- Verifying the Token: When a form is submitted, Laravel compares the token submitted with the form against the token stored in the user’s session. If the tokens match, the request is processed. If they don’t match, the request is rejected.
Implementing CSRF Protection
Automatically Applied Middleware
Laravel automatically applies the CSRF protection middleware to all routes defined in the web
middleware group. This group is usually defined in the routes/web.php
file.
Adding CSRF Tokens to Forms
When creating forms, you need to include the CSRF token. Laravel provides a Blade directive for this:
The @csrf
directive inserts a hidden input field with the CSRF token.
Handling CSRF Tokens in AJAX Requests
If you’re making AJAX requests, you need to ensure the CSRF token is included in the request headers. Here’s an example using jQuery:
You need to include a meta tag in your HTML to store the CSRF token:
Configuring CSRF Token Rotation
For enhanced security, Laravel allows CSRF tokens to rotate after each request. You can enable this in the VerifyCsrfToken
middleware:
Disabling CSRF Protection for Specific Routes
In some cases, such as when developing APIs, you might need to disable CSRF protection for certain routes. This can be done by specifying the routes to exclude in the VerifyCsrfToken
middleware:
Complete Example of CSRF Protection
Now that we went over different parts of CSRF protection, let’s look at a complete example:
This example demonstrates how Laravel protects a simple form from CSRF attacks by verifying the CSRF token embedded in the form.
Common Issues and Troubleshooting
Issue: TokenMismatchException
If you encounter a TokenMismatchException
, it usually means the CSRF token is missing or invalid. Here are some steps to troubleshoot:
- Ensure the
@csrf
directive is included in your forms. - Verify that your AJAX requests include the CSRF token in the headers.
- Check if the session is correctly initialized and does not expire prematurely.
Issue: Session Expiration
If your session expires, the CSRF token will be invalid. Make sure your session lifetime is set appropriately in the config/session.php
configuration file.
Conclusion
CSRF protection is a vital security feature that helps prevent unauthorized actions on behalf of users. Laravel’s built-in CSRF protection is easy to implement and provides a strong defense against CSRF attacks. By following the guidelines in this article, you can ensure your Laravel application is well-protected against these vulnerabilities.