Table of Contents
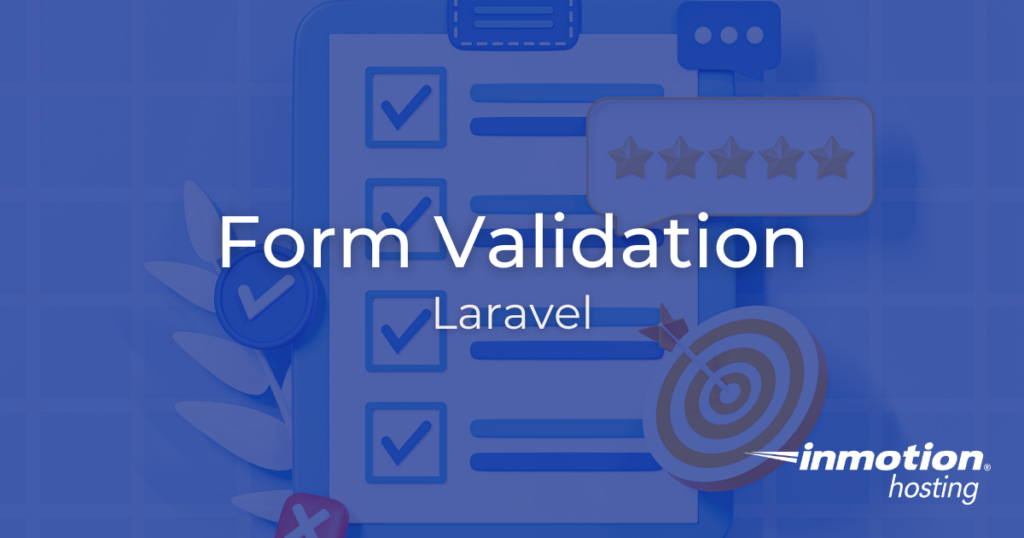
Form validation is a critical aspect of web application development. It ensures that the data submitted by users meets specific criteria before processing. Laravel provides a powerful and easy-to-use validation system that simplifies this process. This article will guide you through implementing and customising form validation in Laravel.
- Introduction to Laravel Form Validation
- Basic Validation Example
- Using Form Request Validation
- Customizing Error Messages
- Validating Arrays
- Conditional Validation
- Custom Validation Rules
- Displaying Validation Errors
- Conclusion
Introduction to Laravel Form Validation
Laravel’s validation system is designed to make validating user input simple and effective. Whether you’re validating simple form inputs or complex nested arrays, Laravel provides all the necessary tools to handle validation efficiently.
Basic Validation Example
Laravel’s most straightforward way to validate a form request is by using the validate
method directly in your controller.
In this example, the validate
method takes an array of validation rules. Laravel automatically redirects the user to the previous page if the validation fails.
Laravel’s Documentation contains all available validation rules and their function.
Using Form Request Validation
For more complex validation logic or when you need to reuse validation rules, it’s best to use Form Request Validation. This involves creating a custom request class.
Step 1: Generate a Form Request class
Step 2: Define validation rules in the generated class
Step 3: Use the Form Request in your controller
Form Requests provide a clean way to organize validation logic, especially in large applications.
Customizing Error Messages
Laravel allows you to customize the error messages returned when validation fails. You can do this using the validation method or Form Request class.
Inline in the controller
In a Form Request Class
Custom messages make your application more user-friendly by providing clearer and more descriptive feedback.
Validating Arrays
When your form inputs are arrays, Laravel handles the validation seamlessly. For example:
In this example, products.*.name
ensures that each product in the array has a name
attribute, and products.*.quantity
checks that the quantity
is an integer greater than zero.
Conditional Validation
Sometimes, you must apply validation rules conditionally based on another field’s value. Laravel offers an elegant way to handle this.
Here, the phone
field is required only if contact_preference
is set to phone
.
Custom Validation Rules
Laravel allows you to define custom validation rules if the built-in ones do not meet your needs.
Create a custom rule
Define the validation logic
Use the custom rule in your application
Custom rules allow you to extend Laravel’s validation capabilities to suit your application’s specific needs.
Displaying Validation Errors
After validating the form, Laravel automatically redirects back to the form with the error messages stored in the session. You can easily display these errors to the user in your Blade view.
This snippet will display a list of all validation errors, providing clear feedback to the user.
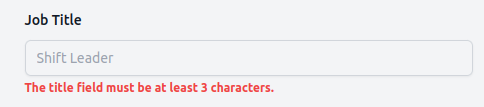
Conclusion
Form validation is essential to any Laravel application, ensuring that the data processed is clean, accurate, and secure. Laravel’s validation system is robust and flexible, making it easy to implement validation logic in your application. Whether using basic validation, custom rules, or conditional logic, Laravel provides all the tools necessary to manage user input effectively.
Following the guidelines and examples in this article, you can confidently handle form validation in your Laravel projects, ensuring a smooth user experience and maintaining the integrity of your application’s data.
Boost your Laravel apps with our specialized Laravel Hosting. Experience faster speeds for your Laravel applications and websites thanks to NVMe storage, server protection, dedicated resources, and optimization tools.
99.99% Uptime
Free SSL
Dedicated IP Address
Developer Tools