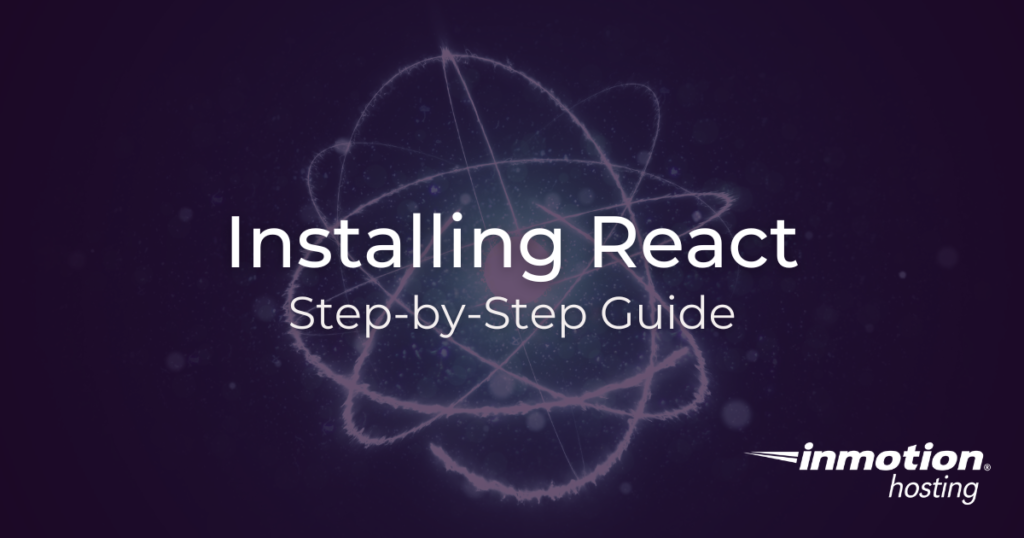
React is a popular JavaScript library for building user interfaces, especially single-page applications where dynamic content updates are essential. This guide will walk you through the updated steps to install React using the latest tools like Vite and Next.js and explain why you should use a framework tailored to your needs.
What Is React?
React, developed by Facebook, is a powerful library that makes building interactive and reusable user interface components easier. Its component-based architecture allows developers to create complex UIs by breaking them into smaller, manageable parts. It is widely used for creating single-page applications (SPAs) that update efficiently without full-page reloads.
Why Use React?
- Component-based: Simplifies UI creation with reusable components.
- Fast rendering: Optimizes performance through the virtual DOM.
- Rich ecosystem: Supported by a strong community and a wide range of libraries.
What You Will Need
Before you begin, make sure you have:
- Node.js: This includes Node Package Manager (NPM), which is necessary for installing React.
- A code editor: Visual Studio Code (VS Code) is a popular choice.
You can check if Node.js is installed by running the following command in your terminal:
node -v
If Node.js isn’t installed, download and install it from the official Node.js website.
We recommend sticking to a VPS Hosting plan or a Dedicated Server, allowing you to install additional software.
Enjoy high-performance, lightning-fast servers with increased security and maximum up-time with our Secure VPS Hosting!
Linux VPS
cPanel or Control Web Panel
Scalable
Website Migration Assistance
Step-by-Step Guide to Installing React
You may still come across tutorials or guides that recommend Create React App (CRA) as a starting point for React projects. However, it is important to note that CRA was deprecated in 2023 due to its outdated build processes and limited capabilities compared to modern tools. Using frameworks like Vite, Next.js, or Remix is now encouraged for new projects.
Step 1: Create a React Application Using Vite
Vite is now a popular choice for React projects due to its fast build times and modern development experience. To create a React app using Vite, follow these steps:
Run the following command in your terminal:
npm create vite@latest my-react-app --template react
Explanation
npm create vite@latest
initializes a new Vite project.my-react-app
is the name of your project, which you can replace with any name you like.--template react
sets up the project specifically for React.
Step 2: Navigate to Your Project Directory
Move into your project folder:
cd my-react-app
Step 3: Install Dependencies
Install all necessary project dependencies:
npm install
Step 4: Start the React Application
Start the development server to see your application running locally:
npm run dev
This command opens your React app in your default browser at http://localhost:5173/
(or a different port if 5173 is in use). You should see the default Vite + React welcome page, indicating your setup is complete.
Step 5: Explore the Project Structure
The Vite project structure will look similar to this:
my-react-app/
├── node_modules/
├── public/
│ └── index.html
├── src/
│ ├── App.css
│ ├── App.jsx
│ ├── main.jsx
│ └── index.css
├── package.json
└── README.md
Key Folders/Files:
src/
: Contains your React components and main code.public/
: Holds static files such asindex.html
.package.json
: Lists dependencies and scripts for your project.
Step 6: Modify Your First Component
Open App.jsx
in your code editor and replace the default content with:
function App() {
return (
<div>
<h1>Hello, React with Vite!</h1>
<p>Welcome to your first React app using Vite.</p>
</div>
);
}
export default App;
Save your changes, and your browser will automatically refresh to display the updated content.
Best Practices for Using React
- Use functional components and hooks: Modern React development favors functional components with hooks (
useState
,useEffect
) for cleaner, more readable code. - Keep components small and modular: Design components to focus on a single responsibility.
- Maintain a clear folder structure: Organize components into subfolders as your app grows.
Choosing the Right React Framework and Build Tools
When deciding how to set up a new React project, it’s crucial to choose the right tool based on your project needs. Here’s an overview of some frameworks and why you might use them, inspired by React’s official recommendations:
- Vite: Build tool that is ideal for simple, fast setups and development with React. It provides an optimized development experience and quick hot reloading.
- Next.js: A full-featured framework for building React applications with server-side rendering, static site generation, and API routes. Use this if you need more advanced routing and server capabilities.
- Remix: Focuses on full-stack web development with a strong emphasis on progressive enhancement and server-side rendering. Consider this for applications that need more robust server interactions.
Each of these frameworks and tools offers a modern, optimized way to start and build React applications with better performance and scalability compared to the now-deprecated CRA.
Conclusion
With the deprecation of Create React App, modern tools like Vite and Next.js provide a more efficient and feature-rich environment for React development. By following this guide, you’ve set up a React app using Vite and learned the essentials for starting your journey in building React applications. Explore these frameworks to find the best fit for your project’s requirements and take your React skills to the next level.
For more tutorials and resources on React, check out our support articles.